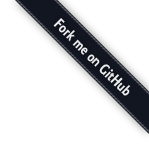
Function Prototype
/* Linear Decay */
/* Type: math */
/* Input arrays: 1 Options: 1 Output arrays: 1 */
/* Inputs: real */
/* Options: period */
/* Outputs: decay */
int ti_decay_start(TI_REAL const *options);
int ti_decay(int size,
TI_REAL const *const *inputs,
TI_REAL const *options,
TI_REAL *const *outputs);
Description
This documentation is still a work in progress. It has omissions, and it probably has errors too. If you see any issues, or have any general feedback, please get in touch.
Decay is a simple function used to propagate signals from the past into the future. It is useful in conjunction with algorithm trading and machine learning functions.
For example, if you have an input array that represents when two moving averages %cross%, it might look like this:
where each 1 represents a day where a crossover occurred. However, it is useful to know about how far back the most recent crossover was, so running that array through decay with a period of 4 produces:
\begin{bmatrix} 0 & 0 & 0 & 1 & .75 & .5 & .25 & 1 & .75 & .5 \end{bmatrix}
See Also
Example Usage
Calling From C
/* Example usage of Linear Decay */
/* Assuming that 'input' is a pre-loaded array of size 'in_size'. */
TI_REAL *inputs[] = {input};
TI_REAL options[] = {5}; /* period */
TI_REAL *outputs[1]; /* decay */
/* Determine how large the output size is for our options. */
const int out_size = in_size - ti_decay_start(options);
/* Allocate memory for output. */
outputs[0] = malloc(sizeof(TI_REAL) * out_size); assert(outputs[0] != 0); /* decay */
/* Run the actual calculation. */
const int ret = ti_decay(in_size, inputs, options, outputs);
assert(ret == TI_OKAY);
Calling From Lua (with Tulip Chart bindings)
-- Example usage of Linear Decay
decay = ti.decay(input, 5)
Example Calculation
period = 5
date | input | decay |
---|---|---|
2005-11-01 | 81.59 | 81.59 |
2005-11-02 | 81.06 | 81.39 |
2005-11-03 | 82.87 | 82.87 |
2005-11-04 | 83.00 | 83.00 |
2005-11-07 | 83.61 | 83.61 |
2005-11-08 | 83.15 | 83.41 |
2005-11-09 | 82.84 | 83.21 |
2005-11-10 | 83.99 | 83.99 |
2005-11-11 | 84.55 | 84.55 |
2005-11-14 | 84.36 | 84.36 |
2005-11-15 | 85.53 | 85.53 |
2005-11-16 | 86.54 | 86.54 |
2005-11-17 | 86.89 | 86.89 |
2005-11-18 | 87.77 | 87.77 |
2005-11-21 | 87.29 | 87.57 |
Chart
Other Indicators
Previous indicator: Chaikins Volatility
Next indicator: Double Exponential Moving Average
Random indicator: Annualized Historical Volatility