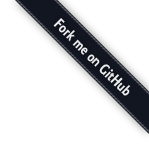
Function Prototype
/* Stochastic Oscillator */
/* Type: indicator */
/* Input arrays: 3 Options: 3 Output arrays: 2 */
/* Inputs: high, low, close */
/* Options: %k period, %k slowing period, %d period */
/* Outputs: stoch_k, stoch_d */
int ti_stoch_start(TI_REAL const *options);
int ti_stoch(int size,
TI_REAL const *const *inputs,
TI_REAL const *options,
TI_REAL *const *outputs);
Description
This documentation is still a work in progress. It has omissions, and it probably has errors too. If you see any issues, or have any general feedback, please get in touch.
The Stochastic Oscillator indicator calculates two values, %k
and %d
.
It takes three options: the %k period
n
, the %k slowing period
m
, and the %d period
p
.
The calculation is as follows:
$$max_{t} = maximum(high_{t}, high_{t-1}, ..., high_{t-n+1}) $$
$$min_{t} = minimum(low_{t}, low_{t-1}, ..., low_{t-n+1}) $$
$$fastk_{t} = 100 \frac{close_{t} - min_{t}}{max_{t} - min_{t}}$$
Then the first output, %k
, is a Simple Moving Average of period m
applied to fastk
. The second
output, %d
, is a Simple Moving Average of period p
applied to %k
.
$$k_{t} = \frac{1}{m} \sum_{i=0}^{m-1} fastk_{t-i}$$
$$d_{t} = \frac{1}{p} \sum_{i=0}^{p-1} k_{t-i}$$
See Also
References
- Murphy, J. (1999) Technical Analysis of the Financial Markets
- Achelis, S. (2000) Technical Analysis from A to Z, 2nd Edition
Example Usage
Calling From C
/* Example usage of Stochastic Oscillator */
/* Assuming that 'high', 'low', and 'close' are pre-loaded arrays of size 'in_size'. */
TI_REAL *inputs[] = {high, low, close};
TI_REAL options[] = {5, 3, 3}; /* %k period, %k slowing period, %d period */
TI_REAL *outputs[2]; /* stoch_k, stoch_d */
/* Determine how large the output size is for our options. */
const int out_size = in_size - ti_stoch_start(options);
/* Allocate memory for each output. */
outputs[0] = malloc(sizeof(TI_REAL) * out_size); assert(outputs[0] != 0); /* stoch_k */
outputs[1] = malloc(sizeof(TI_REAL) * out_size); assert(outputs[1] != 0); /* stoch_d */
/* Run the actual calculation. */
const int ret = ti_stoch(in_size, inputs, options, outputs);
assert(ret == TI_OKAY);
Calling From Lua (with Tulip Chart bindings)
-- Example usage of Stochastic Oscillator
stoch_k, stoch_d = ti.stoch(high, low, close, 5, 3, 3)
Example Calculation
%k period = 5, %k slowing period = 3, %d period = 3
date | high | low | close | stoch_k | stoch_d |
---|---|---|---|---|---|
2005-11-01 | 82.15 | 81.29 | 81.59 | ||
2005-11-02 | 81.89 | 80.64 | 81.06 | ||
2005-11-03 | 83.03 | 81.31 | 82.87 | ||
2005-11-04 | 83.30 | 82.65 | 83.00 | ||
2005-11-07 | 83.85 | 83.07 | 83.61 | ||
2005-11-08 | 83.90 | 83.11 | 83.15 | ||
2005-11-09 | 83.33 | 82.49 | 82.84 | ||
2005-11-10 | 84.30 | 82.30 | 83.99 | ||
2005-11-11 | 84.84 | 84.15 | 84.55 | 77.39 | 75.70 |
2005-11-14 | 85.00 | 84.11 | 84.36 | 83.13 | 78.01 |
2005-11-15 | 85.90 | 84.03 | 85.53 | 84.87 | 81.79 |
2005-11-16 | 86.58 | 85.39 | 86.54 | 88.36 | 85.45 |
2005-11-17 | 86.98 | 85.76 | 86.89 | 95.25 | 89.49 |
2005-11-18 | 88.00 | 87.17 | 87.77 | 96.74 | 93.45 |
2005-11-21 | 87.87 | 87.01 | 87.29 | 91.09 | 94.36 |
Chart
Other Indicators
Previous indicator: Standard Error Over Period
Next indicator: Stochastic RSI
Random indicator: Vector Square Root
Copyright © 2016-2024 Tulip Charts LLC. All Rights Reserved.