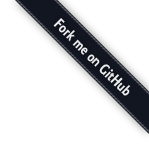
Function Prototype
/* Linear Regression */
/* Type: overlay */
/* Input arrays: 1 Options: 1 Output arrays: 1 */
/* Inputs: real */
/* Options: period */
/* Outputs: linreg */
int ti_linreg_start(TI_REAL const *options);
int ti_linreg(int size,
TI_REAL const *const *inputs,
TI_REAL const *options,
TI_REAL *const *outputs);
Description
This documentation is still a work in progress. It has omissions, and it probably has errors too. If you see any issues, or have any general feedback, please get in touch.
The Linear Regression is a smoothing functions that works by preforming linear least squares regression over a moving window. It then uses the linear model to predict the value for the current bar.
It takes one parameter, the period n
. Larger values for n
will have a
greater smoothing effect on the input data but will also create more lag.
It is calculated as:
$$\overline{x} = \frac{1}{n} \sum_{i=1}^{n} i$$
$$\overline{y}_{t} = \frac{1}{n} \sum_{i=0}^{n-1} in_{t-i}$$
$$\widehat{\beta}_{t} =
\frac
{\sum_{i=1}^{n}(i-\overline{x})(in_{(t-n+i)}-\overline{y}_{t})}
{\sum_{i=1}^{n}(i-\overline{x})^{2}}$$
$$\widehat{\alpha}_{t} = \overline{y}_{t} - \widehat{\beta}_{t}\overline{x}$$
$$linreg_{t} = \widehat{\alpha}_{t} + \widehat{\beta}_{t} n$$
See Also
References
- Kaufman, Perry J. (2013) Trading Systems and Methods
- Chande, Tushar S. (1994) The New Technical Trader
- Wikipedia: Simple Linear Regression
Example Usage
Calling From C
/* Example usage of Linear Regression */
/* Assuming that 'input' is a pre-loaded array of size 'in_size'. */
TI_REAL *inputs[] = {input};
TI_REAL options[] = {5}; /* period */
TI_REAL *outputs[1]; /* linreg */
/* Determine how large the output size is for our options. */
const int out_size = in_size - ti_linreg_start(options);
/* Allocate memory for output. */
outputs[0] = malloc(sizeof(TI_REAL) * out_size); assert(outputs[0] != 0); /* linreg */
/* Run the actual calculation. */
const int ret = ti_linreg(in_size, inputs, options, outputs);
assert(ret == TI_OKAY);
Calling From Lua (with Tulip Chart bindings)
-- Example usage of Linear Regression
linreg = ti.linreg(input, 5)
Example Calculation
period = 5
date | input | linreg |
---|---|---|
2005-11-01 | 81.59 | |
2005-11-02 | 81.06 | |
2005-11-03 | 82.87 | |
2005-11-04 | 83.00 | |
2005-11-07 | 83.61 | 83.62 |
2005-11-08 | 83.15 | 83.72 |
2005-11-09 | 82.84 | 83.11 |
2005-11-10 | 83.99 | 83.56 |
2005-11-11 | 84.55 | 84.17 |
2005-11-14 | 84.36 | 84.60 |
2005-11-15 | 85.53 | 85.40 |
2005-11-16 | 86.54 | 86.21 |
2005-11-17 | 86.89 | 86.95 |
2005-11-18 | 87.77 | 87.85 |
2005-11-21 | 87.29 | 87.75 |
Chart
Other Indicators
Previous indicator: Lag
Next indicator: Linear Regression Intercept
Random indicator: Fisher Transform
Copyright © 2016-2024 Tulip Charts LLC. All Rights Reserved.